Simplified File Downloads on Shared Hosting with PHP and cURL: A Step-by-Step Guide
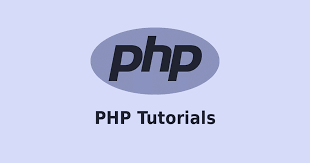
In the world of web development and shared hosting, there often comes a time when you need to download files from the internet to your hosting server. While the wget
command is a powerful tool for this purpose on a dedicated server, shared hosting environments may have restrictions that limit its usage. So, what can you do when you’re working with shared hosting and need to download files programmatically? The answer lies in crafting a PHP script to handle the task seamlessly.
In this guide, we’ll walk you through the process of creating a PHP script that can download files from the internet to your shared hosting server. We’ll use the cURL library, which is commonly available on most shared hosting platforms.
Why Use PHP and cURL on Shared Hosting?
Shared hosting environments often impose restrictions on the usage of system-level commands like wget
. However, PHP is typically allowed and provides a versatile means to interact with web resources. By utilizing the cURL library within PHP, you can easily retrieve files from remote servers and store them on your shared hosting account.
Prerequisites
Before we dive into the PHP script, ensure that you have the following in place:
- Access to a shared hosting account.
- Basic knowledge of PHP programming.
The PHP Script
Below is the PHP script that you can use to download files from the internet to your shared hosting server: (wget
alternative shared hosting with php script. How to Download Files to Server Using PHP Script. How to download files from client to server.)
<?php
// URL of the file you want to download
$fileUrl = 'https://example.com/path/to/your/file.ext';
// Destination path on your server where you want to save the downloaded file
$destination = 'path/to/destination/file.ext';
// Use cURL to download the file
$ch = curl_init($fileUrl);
$fp = fopen($destination, 'w');
curl_setopt($ch, CURLOPT_FILE, $fp);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error: ' . curl_error($ch);
} else {
echo 'File downloaded successfully.';
}
curl_close($ch);
fclose($fp);
?>
Let’s break down how this script works:
- $fileUrl: Set this variable to the URL of the file you want to download.
- $destination: Specify the path on your shared hosting server where you want to save the downloaded file.
The script uses cURL to perform the download, creating a cURL handle with curl_init()
, opening a file handle for writing with fopen()
, and configuring cURL options with curl_setopt()
. It then executes the cURL request, checks for errors, and provides feedback on the download status.
Using the Script
To use this script:
- Replace
'https://example.com/path/to/your/file.ext'
with the actual URL of the file you want to download. - Replace
'path/to/destination/file.ext'
with the desired path on your shared hosting server where you want to save the downloaded file. - Upload the script to your shared hosting environment using FTP or your hosting control panel.
- Access the script through a web browser or execute it via command-line PHP, depending on your hosting environment.
Conclusion
When working in a shared hosting environment, downloading files programmatically doesn’t have to be a headache. By creating a simple PHP script that leverages the cURL library, you can effortlessly download files from the internet to your shared hosting server. This approach ensures compatibility with the constraints of shared hosting while empowering you to automate essential tasks effectively.
Now that you have this handy PHP script in your toolkit, you can streamline file downloads on your shared hosting account and make your web development tasks even more efficient. Happy coding!